Code: Alles auswählen
library ieee;
use ieee.std_logic_1164.all;
entity fulladder is
port (
a, b, c: in std_logic;
s, u: out std_logic
);
end;
architecture behaviour of fulladder is
begin
s <= a xor b xor c;
u <= (a and b) or ((a or b) and c);
end;
library ieee;
use ieee.std_logic_1164.all;
entity ripplecarryadder32 is
port (
s: out std_logic_vector (31 downto 0);
b, a: in std_logic_vector (31 downto 0)
);
end;
architecture behaviour of ripplecarryadder32 is
component fulladder
port (
a, b, c: in std_logic;
s, u: out std_logic
);
end component;
signal c : std_logic_vector (31 downto 0);
signal u : std_logic_vector (31 downto 0);
begin
c(0) <= '0';
add1: fulladder PORT MAP (c=>c(0),b=>b(0),a=>a(0),s=>s(0),u=>u(0));
l1:
for i in 1 to 31 generate
sn: fulladder PORT MAP (c=>u(i-1),b=>b(i),a=>a(i),s=>s(i),u=>u(i));
end generate;
end;
library ieee;
use ieee.std_logic_1164.all;
entity srlatch is
port (
q: out std_logic;
r, s: in std_logic
);
end;
architecture behaviour of srlatch is
signal q1, q2: std_logic;
begin
q1 <= s when q1 = 'U' else (q2 nor s);
q2 <= not s when q2 = 'U' else (q1 nor r);
q <= q1;
end;
library ieee;
use ieee.std_logic_1164.all;
entity csrlatch is
port (
q: out std_logic;
r, s, c: in std_logic
);
end;
architecture behaviour of csrlatch is
component srlatch
port (
q: out std_logic;
r, s: in std_logic
);
end component;
signal s1, r1 : std_logic;
begin
sn: srlatch PORT MAP (s=>s1, r=>r1, q=>q);
s1 <= c and s;
r1 <= c and r;
end;
library ieee;
use ieee.std_logic_1164.all;
entity dlatch is
port (
q: out std_logic;
d, c: in std_logic
);
end;
architecture behaviour of dlatch is
component csrlatch
port (
q: out std_logic;
s, r, c: in std_logic
);
end component;
signal s1, r1 : std_logic;
begin
sn: csrlatch PORT MAP (s=>s1, r=>r1, c=>c, q=>q);
s1 <= d;
r1 <= not d;
end;
library ieee;
use ieee.std_logic_1164.all;
entity dmsff is
port (
q: out std_logic;
d, c: in std_logic
);
end;
architecture behaviour of dmsff is
component dlatch
port (
q: out std_logic;
d, c: in std_logic
);
end component;
signal c1, c2 : std_logic;
signal q1 : std_logic;
begin
sn1: dlatch PORT MAP (d=>d, c=>c1, q=>q1);
sn2: dlatch PORT MAP (d=>q1, c=>c2, q=>q);
c1 <= c;
c2 <= not c;
end;
library ieee;
use ieee.std_logic_1164.all;
entity reg32 is
port (
c: in std_logic;
q: out std_logic_vector (31 downto 0);
d: in std_logic_vector (31 downto 0)
);
end;
architecture behaviour of reg32 is
component dmsff
port (
q: out std_logic;
d, c: in std_logic
);
end component;
begin
l1:
for i in 0 to 31 generate
sn: dmsff PORT MAP (q=>q(i), d=>d(i), c=>c);
end generate;
end;
library ieee;
use ieee.std_logic_1164.all;
entity counter32 is
port (
q: inout std_logic_vector (31 downto 0)
);
end;
architecture behaviour of counter32 is
component reg32
port (
c: in std_logic;
q: out std_logic_vector (31 downto 0);
d: in std_logic_vector (31 downto 0)
);
end component;
component ripplecarryadder32
port (
s: out std_logic_vector (31 downto 0);
b, a: in std_logic_vector (31 downto 0)
);
end component;
signal d: std_logic_vector (31 downto 0);
signal b: std_logic_vector (31 downto 0);
signal a: std_logic_vector (31 downto 0);
signal c: std_logic;
begin
sn1: reg32 PORT MAP (q=>b, d=>d, c=>c);
sn2: ripplecarryadder32 PORT MAP (b=>b, a=>a, s=>d);
a <= ('0','0','0','0','0','0','0','0','0','0','0','0','0','0','0','0','0','0','0','0','0','0','0','0','0','0','0','0','0','0','0','1') after 0 ns;
q <= d;
--b <= ('0','0','0','0','0','0','0','0','0','0','0','0','0','0','0','0','0','0','0','0','0','0','0','0','0','0','0','0','0','0','0','0') after 0 ns;
--d <= ('0','0','0','0','0','0','0','0','0','0','0','0','0','0','0','0','0','0','0','0','0','0','0','0','0','0','0','0','0','0','0','0') after 0 ns;
c <= '0' after 0 ns, '1' after 1 ns, '0' after 2 ns, '1' after 3 ns, '0' after 4 ns, '1' after 5 ns, '0' after 6 ns, '1' after 7 ns;
end;
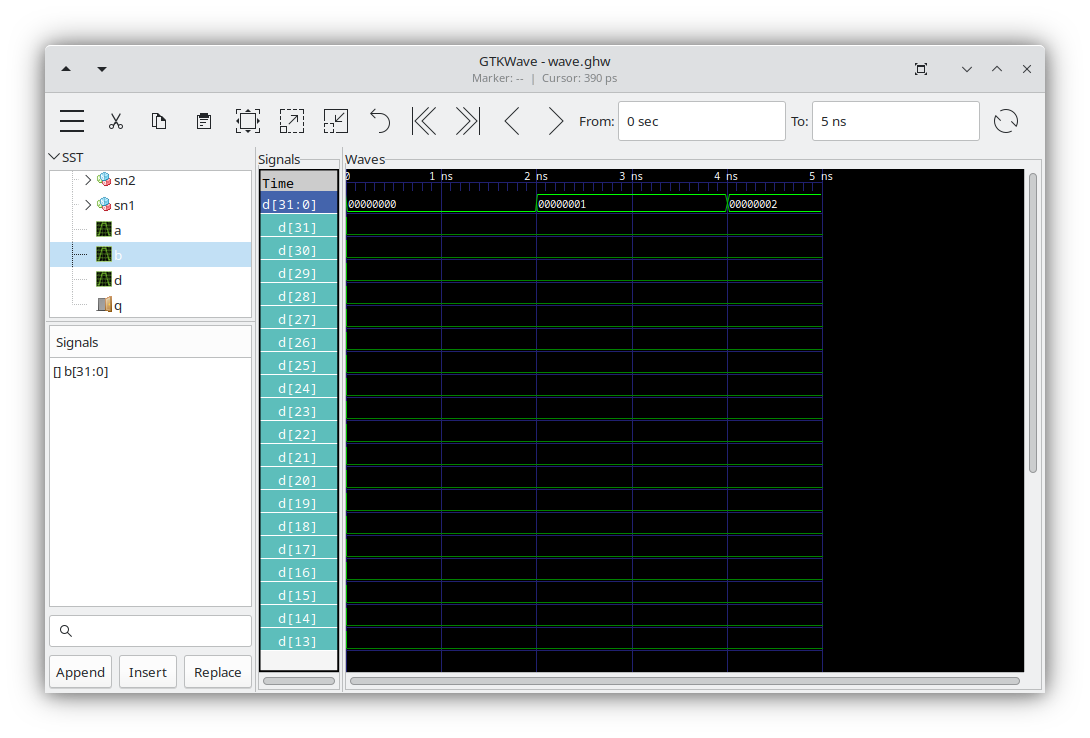
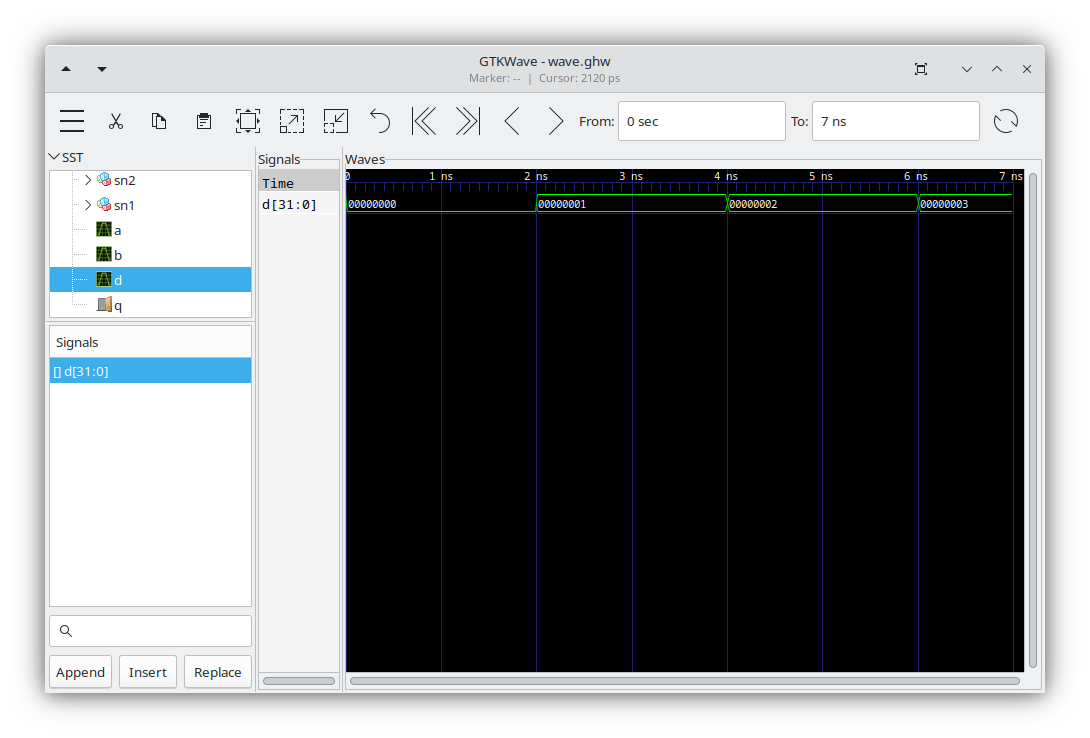

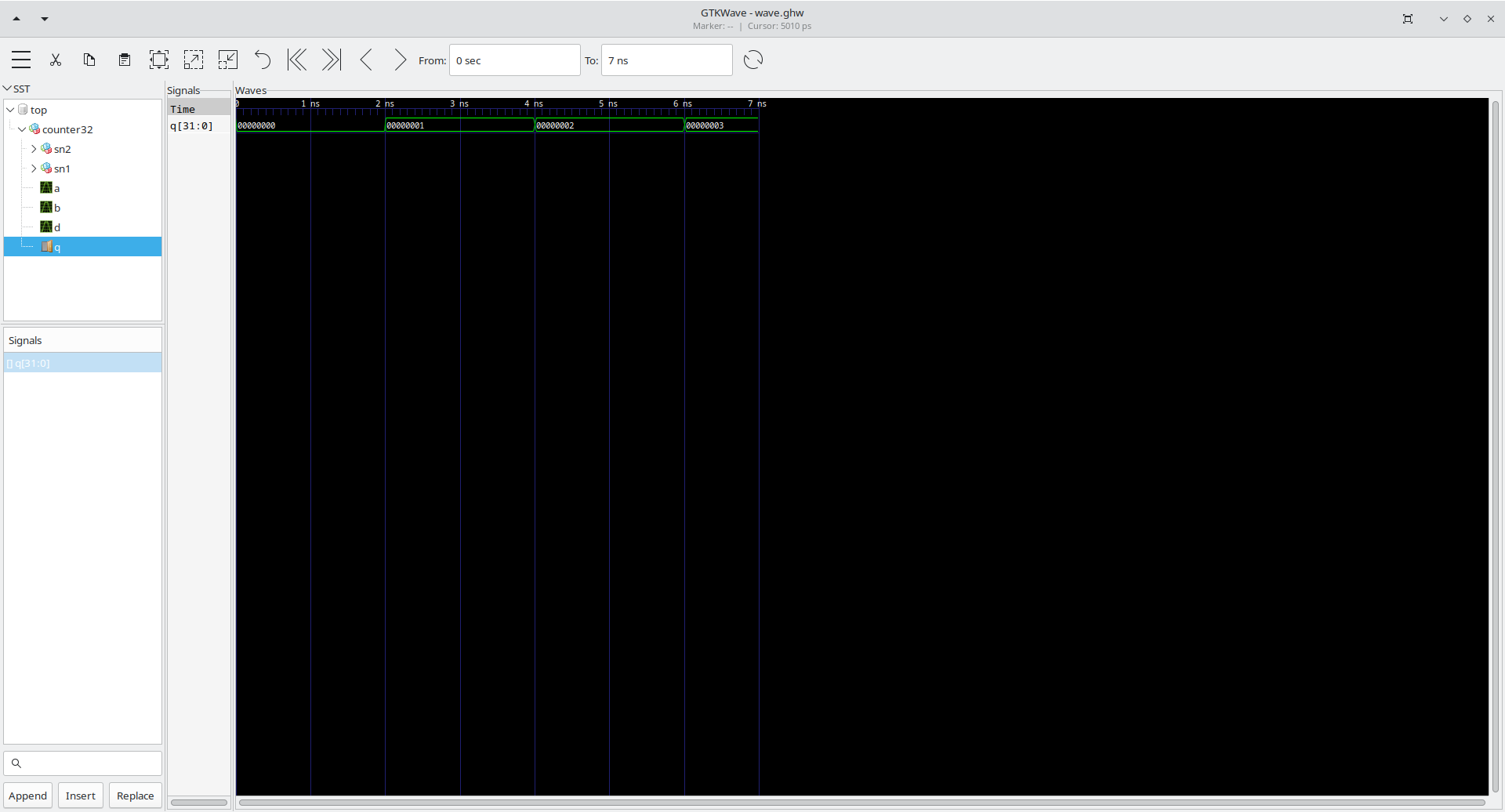
Code: Alles auswählen
library ieee;
use ieee.std_logic_1164.all;
entity fulladder is
port (
a, b, c: in std_logic;
s, u: out std_logic
);
end;
architecture behaviour of fulladder is
begin
s <= a xor b xor c;
u <= (a and b) or ((a or b) and c);
end;
library ieee;
use ieee.std_logic_1164.all;
entity ripplecarryadder32 is
port (
s: out std_logic_vector (31 downto 0);
b, a: in std_logic_vector (31 downto 0)
);
end;
architecture behaviour of ripplecarryadder32 is
component fulladder
port (
a, b, c: in std_logic;
s, u: out std_logic
);
end component;
signal c : std_logic_vector (31 downto 0);
signal u : std_logic_vector (31 downto 0);
begin
c(0) <= '0';
add1: fulladder PORT MAP (c=>c(0),b=>b(0),a=>a(0),s=>s(0),u=>u(0));
l1:
for i in 1 to 31 generate
sn: fulladder PORT MAP (c=>u(i-1),b=>b(i),a=>a(i),s=>s(i),u=>u(i));
end generate;
end;
library ieee;
use ieee.std_logic_1164.all;
entity srlatch is
port (
q: out std_logic;
r, s: in std_logic
);
end;
architecture behaviour of srlatch is
signal q1, q2: std_logic;
begin
q1 <= s when q1 = 'U' else (q2 nor s);
q2 <= not s when q2 = 'U' else (q1 nor r);
q <= q1;
end;
library ieee;
use ieee.std_logic_1164.all;
entity csrlatch is
port (
q: out std_logic;
r, s, c: in std_logic
);
end;
architecture behaviour of csrlatch is
component srlatch
port (
q: out std_logic;
r, s: in std_logic
);
end component;
signal s1, r1 : std_logic;
begin
sn: srlatch PORT MAP (s=>s1, r=>r1, q=>q);
s1 <= c and s;
r1 <= c and r;
end;
library ieee;
use ieee.std_logic_1164.all;
entity dlatch is
port (
q: out std_logic;
d, c: in std_logic
);
end;
architecture behaviour of dlatch is
component csrlatch
port (
q: out std_logic;
s, r, c: in std_logic
);
end component;
signal s1, r1 : std_logic;
begin
sn: csrlatch PORT MAP (s=>s1, r=>r1, c=>c, q=>q);
s1 <= d;
r1 <= not d;
end;
library ieee;
use ieee.std_logic_1164.all;
entity dmsff is
port (
q: out std_logic;
d, c: in std_logic
);
end;
architecture behaviour of dmsff is
component dlatch
port (
q: out std_logic;
d, c: in std_logic
);
end component;
signal c1, c2 : std_logic;
signal q1 : std_logic;
begin
sn1: dlatch PORT MAP (d=>d, c=>c1, q=>q1);
sn2: dlatch PORT MAP (d=>q1, c=>c2, q=>q);
c1 <= c;
c2 <= not c;
end;
library ieee;
use ieee.std_logic_1164.all;
entity reg32 is
port (
c: in std_logic;
q: out std_logic_vector (31 downto 0);
d: in std_logic_vector (31 downto 0)
);
end;
architecture behaviour of reg32 is
component dmsff
port (
q: out std_logic;
d, c: in std_logic
);
end component;
begin
l1:
for i in 0 to 31 generate
sn: dmsff PORT MAP (q=>q(i), d=>d(i), c=>c);
end generate;
end;
library ieee;
use ieee.std_logic_1164.all;
entity counter32 is
port (
q: inout std_logic_vector (31 downto 0)
);
end;
architecture behaviour of counter32 is
component reg32
port (
c: in std_logic;
q: out std_logic_vector (31 downto 0);
d: in std_logic_vector (31 downto 0)
);
end component;
component ripplecarryadder32
port (
s: out std_logic_vector (31 downto 0);
b, a: in std_logic_vector (31 downto 0)
);
end component;
signal d: std_logic_vector (31 downto 0);
signal b: std_logic_vector (31 downto 0);
signal a: std_logic_vector (31 downto 0);
signal c: std_logic;
begin
sn1: reg32 PORT MAP (q=>b, d=>d, c=>c);
sn2: ripplecarryadder32 PORT MAP (b=>b, a=>a, s=>d);
a <= ('0','0','0','0','0','0','0','0','0','0','0','0','0','0','0','0','0','0','0','0','0','0','0','0','0','0','0','0','0','0','0','1') after 0 ns;
q <= d;
--b <= ('0','0','0','0','0','0','0','0','0','0','0','0','0','0','0','0','0','0','0','0','0','0','0','0','0','0','0','0','0','0','0','0') after 0 ns;
--d <= ('0','0','0','0','0','0','0','0','0','0','0','0','0','0','0','0','0','0','0','0','0','0','0','0','0','0','0','0','0','0','0','0') after 0 ns;
c <= '0' after 0 ns, '1' after 1 ns, '0' after 2 ns, '1' after 3 ns, '0' after 4 ns, '1' after 5 ns, '0' after 6 ns, '1' after 7 ns;
end;
Das Problem liegt im Latch selber - am Anfang ist der Zustand 'U'. Das muss man gross schreiben - also Undefined. Und da
Code: Alles auswählen
q1 <= (q2 nor s);
q2 <= (q1 nor r);
Ich habe das so gemacht. Damit geht es
Code: Alles auswählen
architecture behaviour of srlatch is
signal q1, q2: std_logic;
begin
q1 <= s when q1 = 'U' else (q2 nor s);
q2 <= not s when q2 = 'U' else (q1 nor r);
q <= q1;
end;
Der Schritt vom Zähler zum MIPS geht schnell. Weil, jetzt brauchen wir ein WE - an dem Register
Dann müssen wir - schnell einen Multiplexer schreiben, oder ein auswählendes Schaltnetz - dann müssen wir mit den Registern wieder wie vorher mit
Code: Alles auswählen
FOR GENERATE