
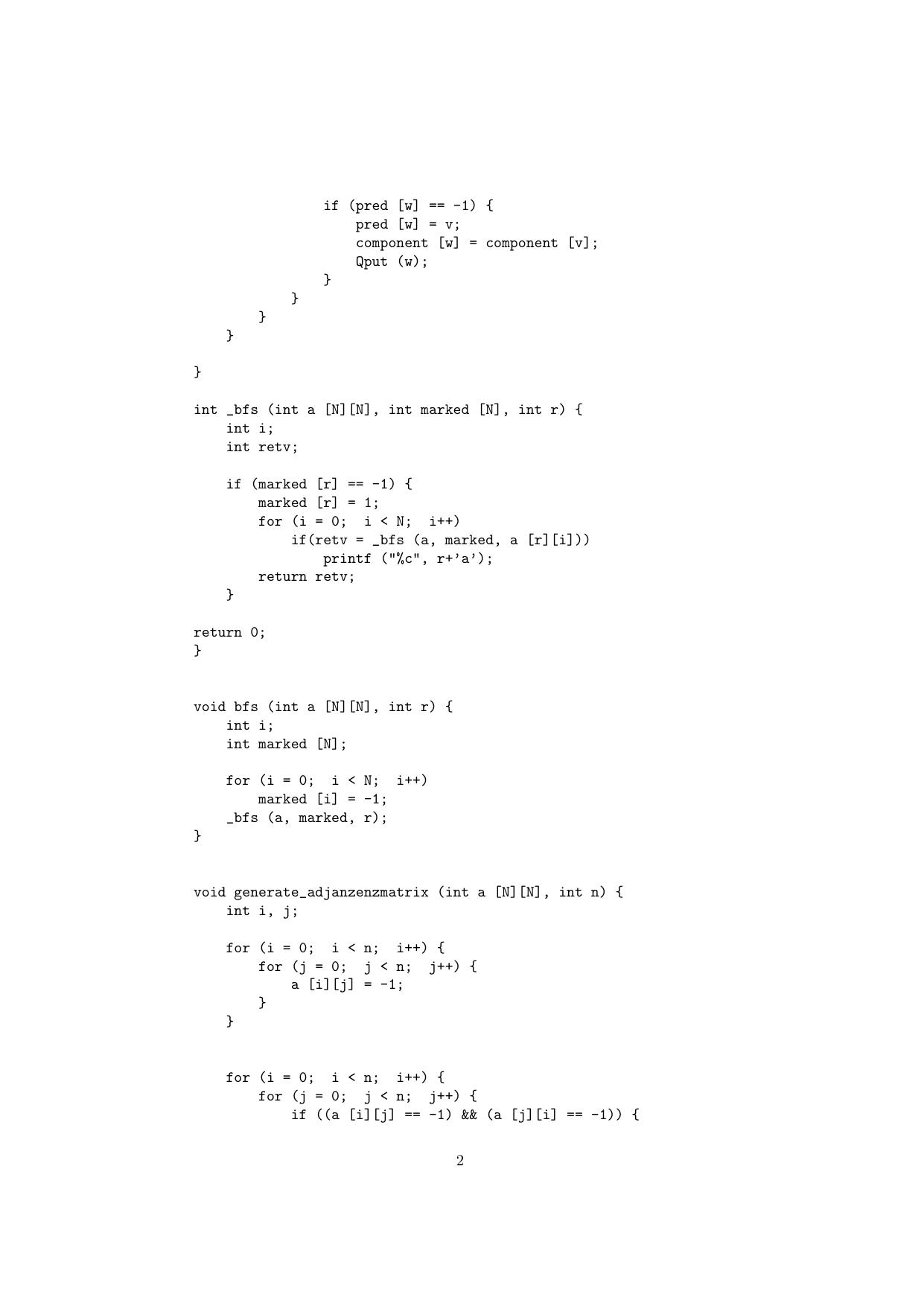
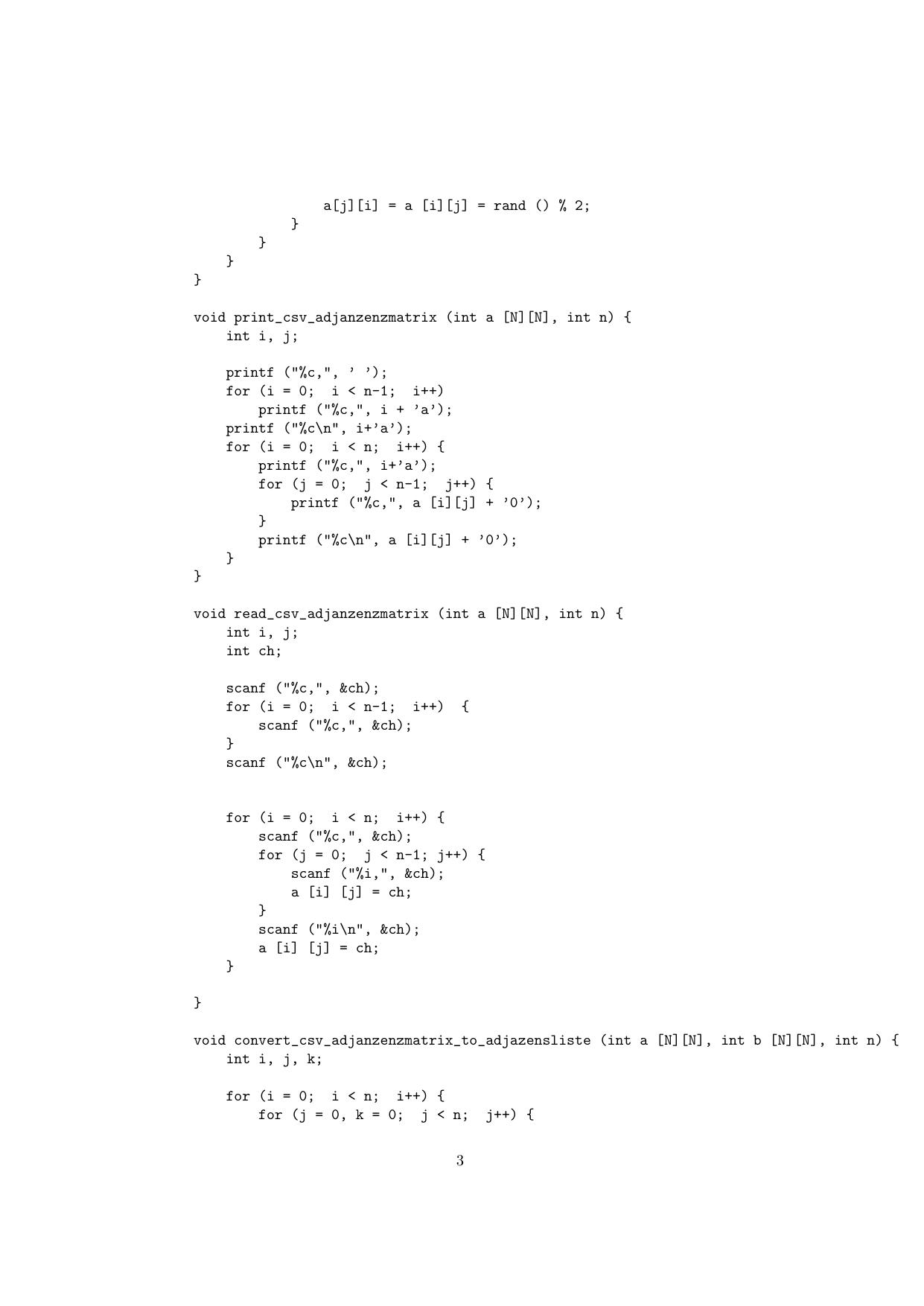
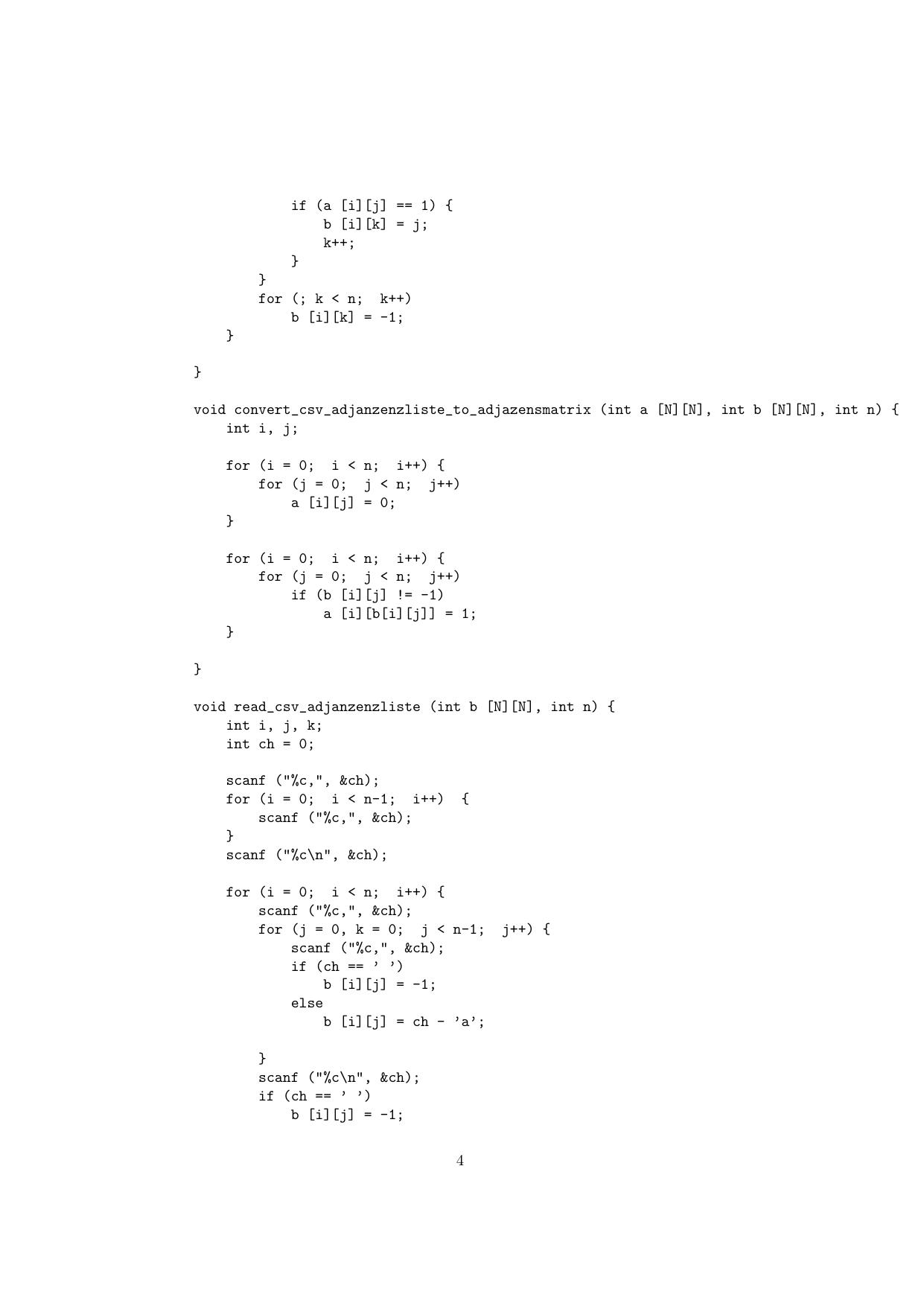
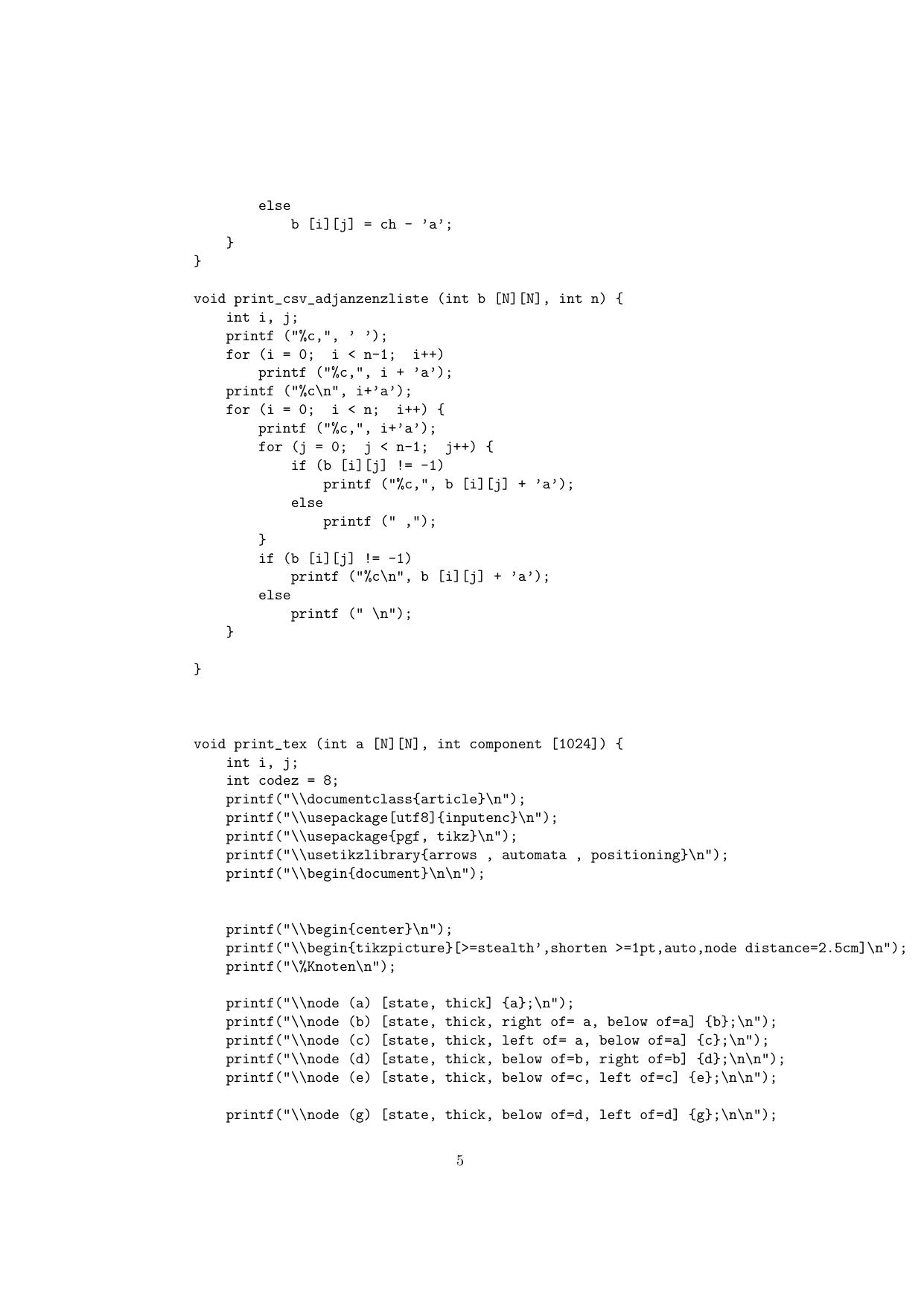

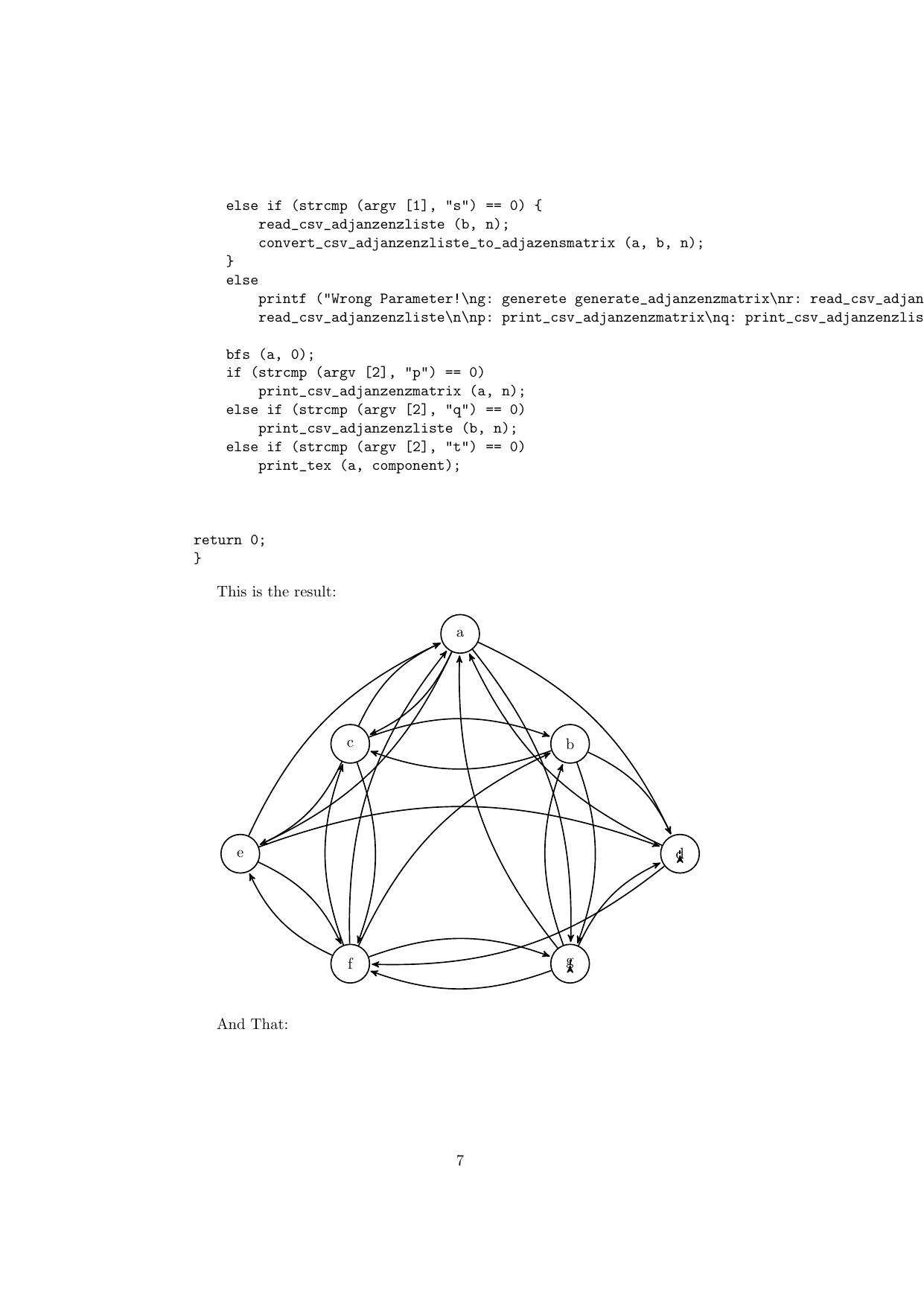
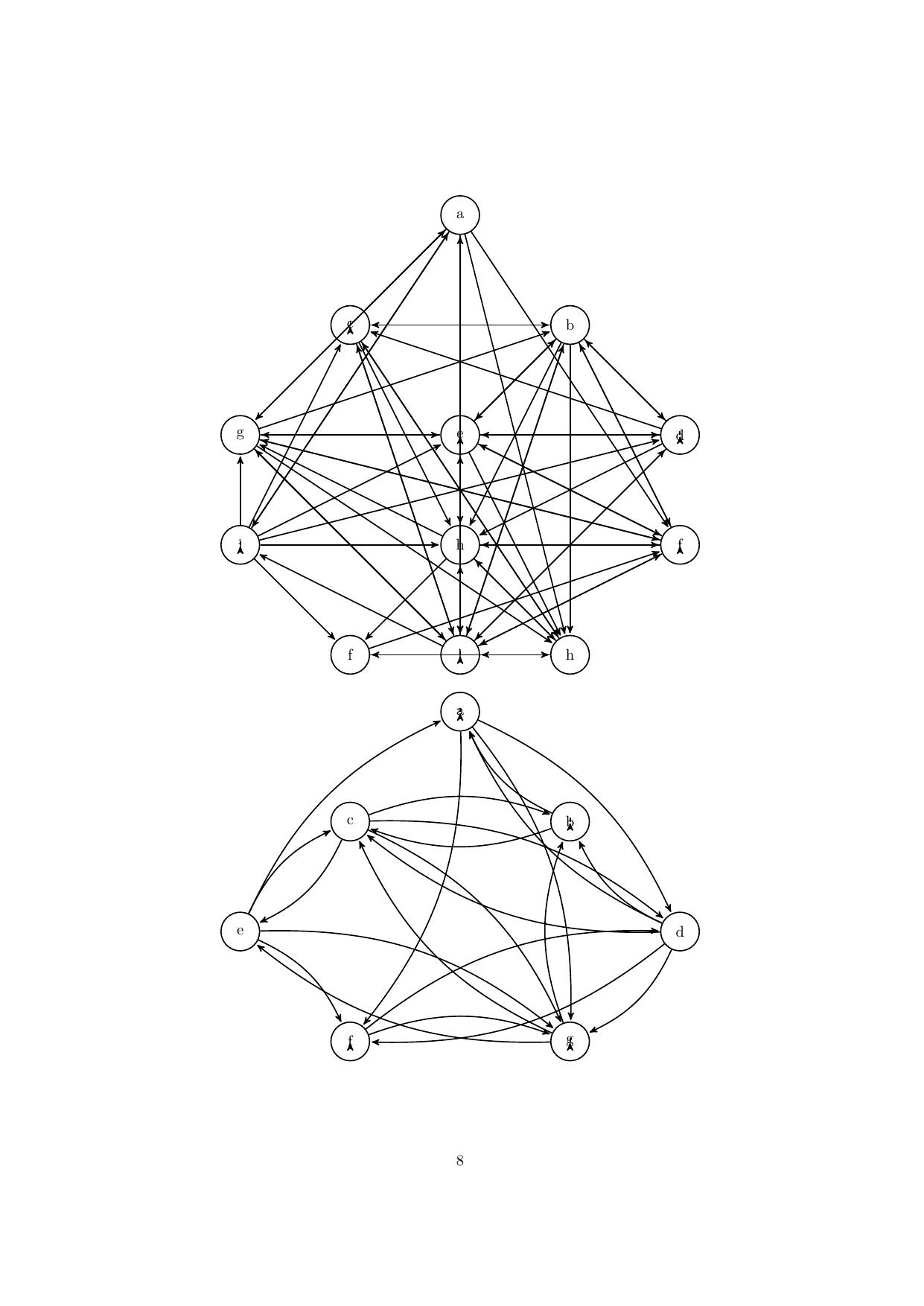
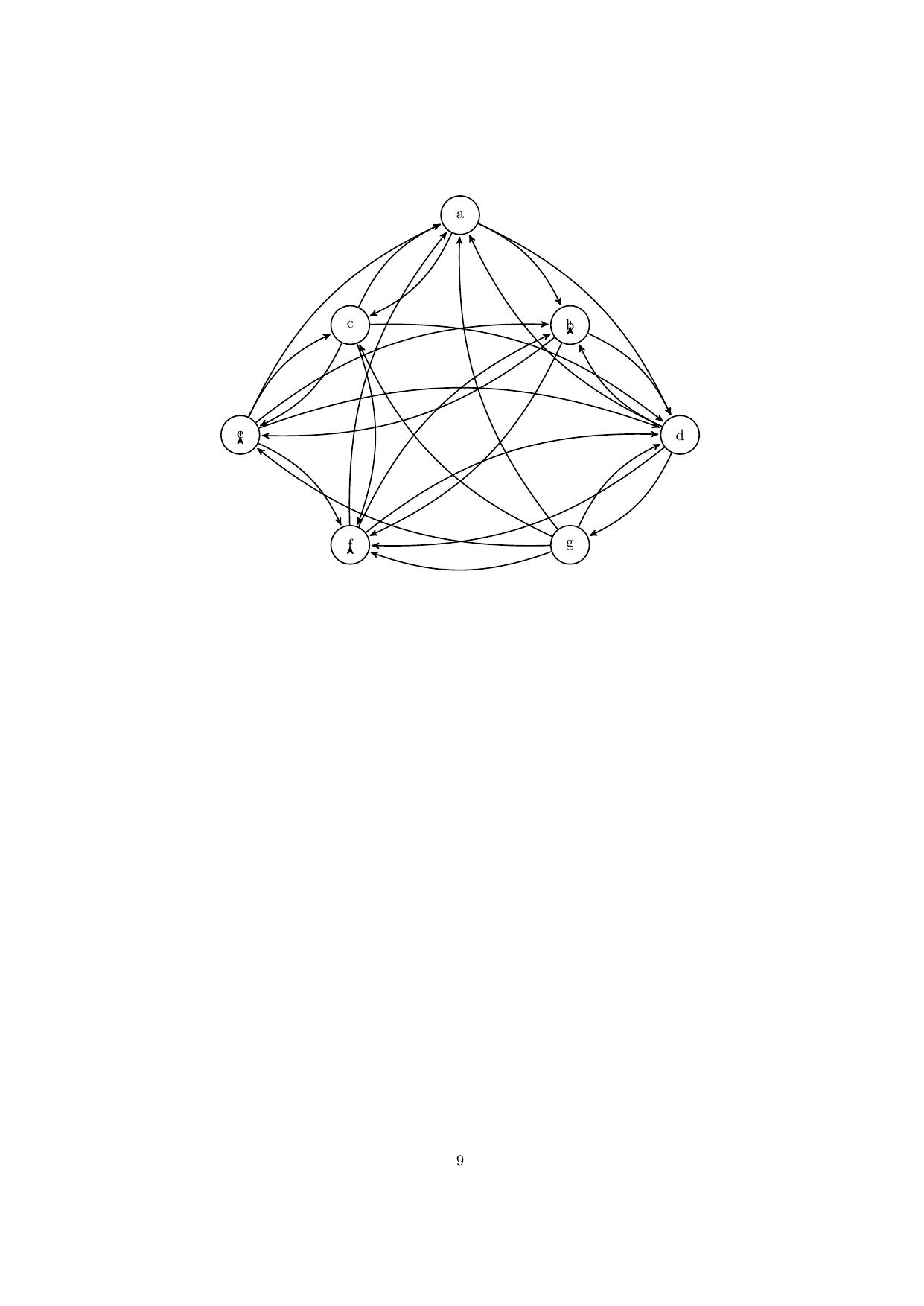
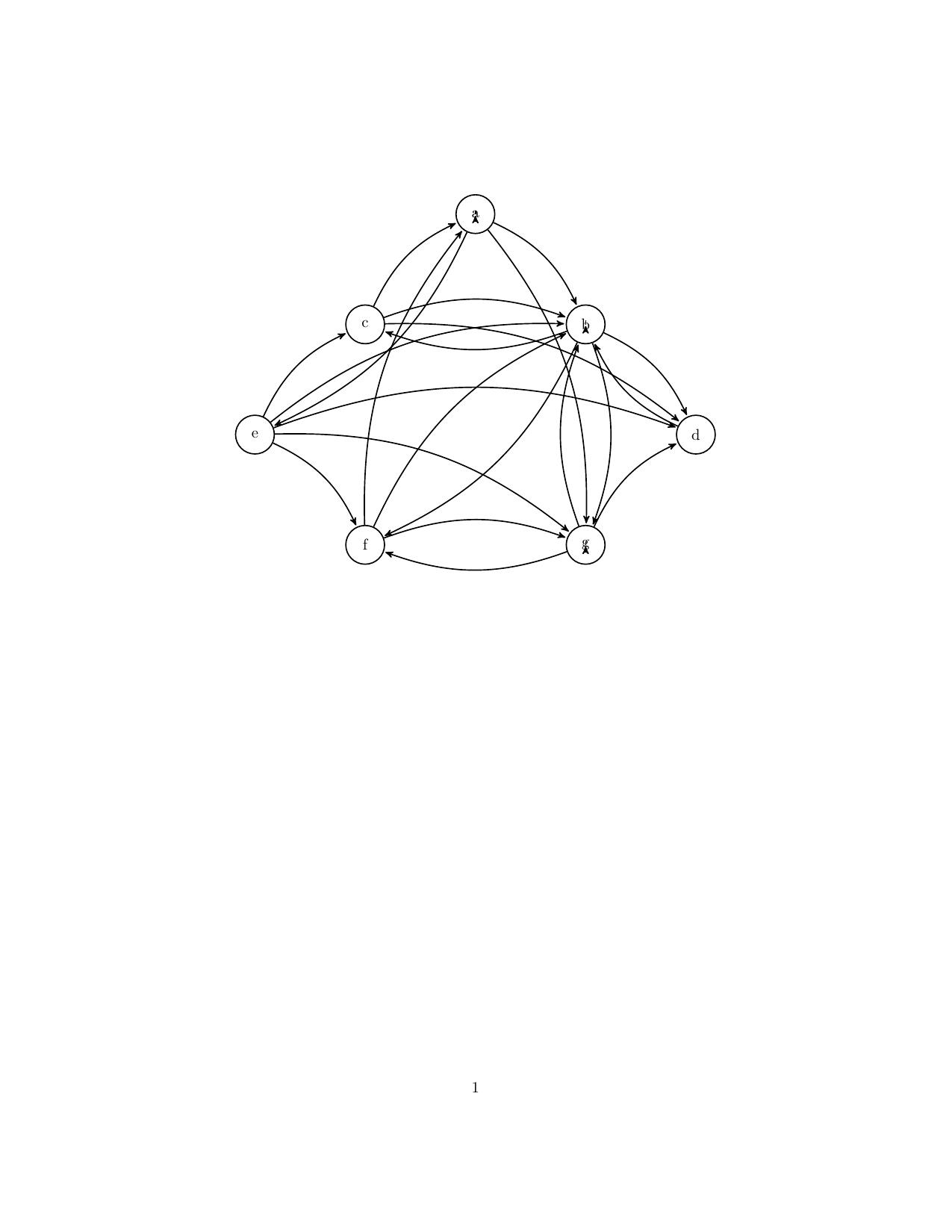
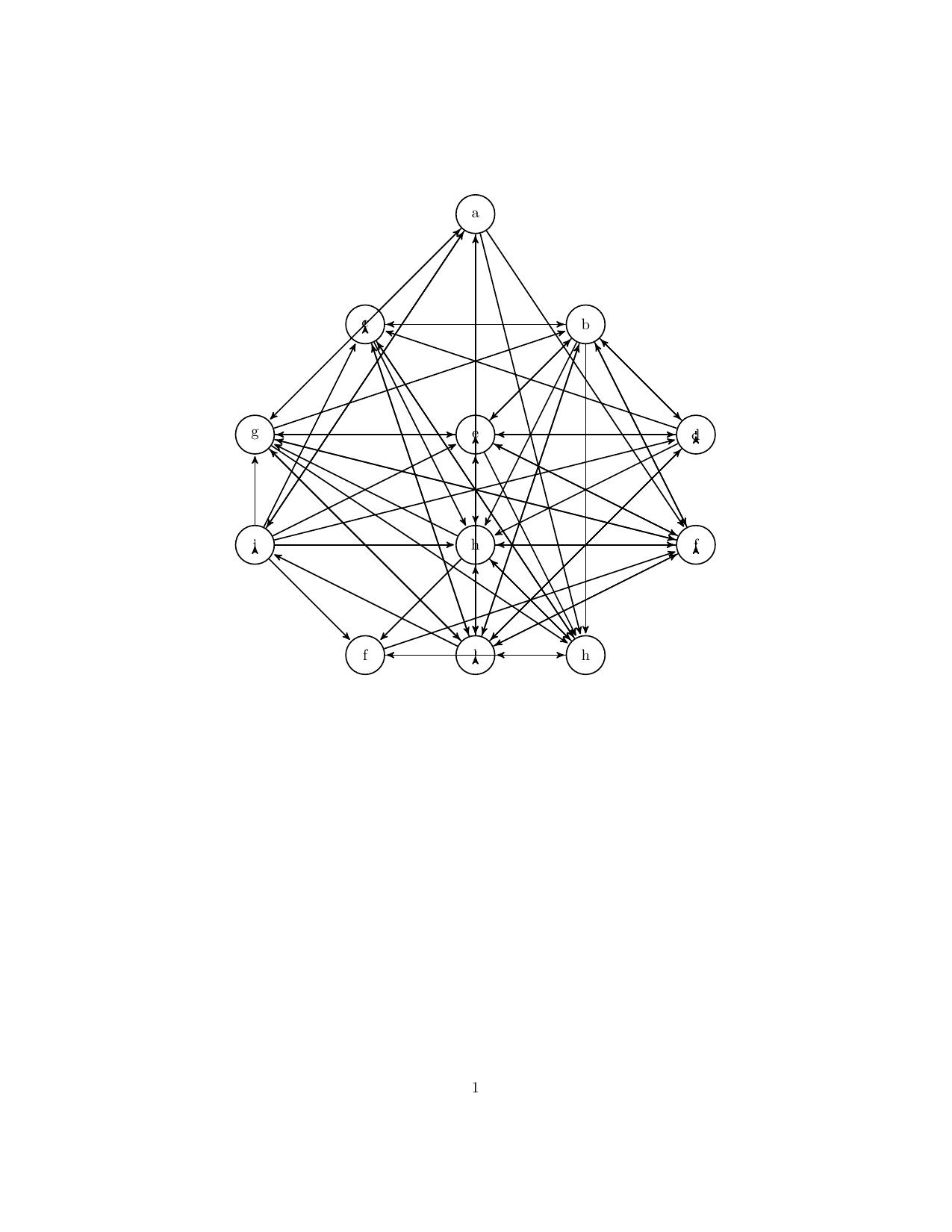
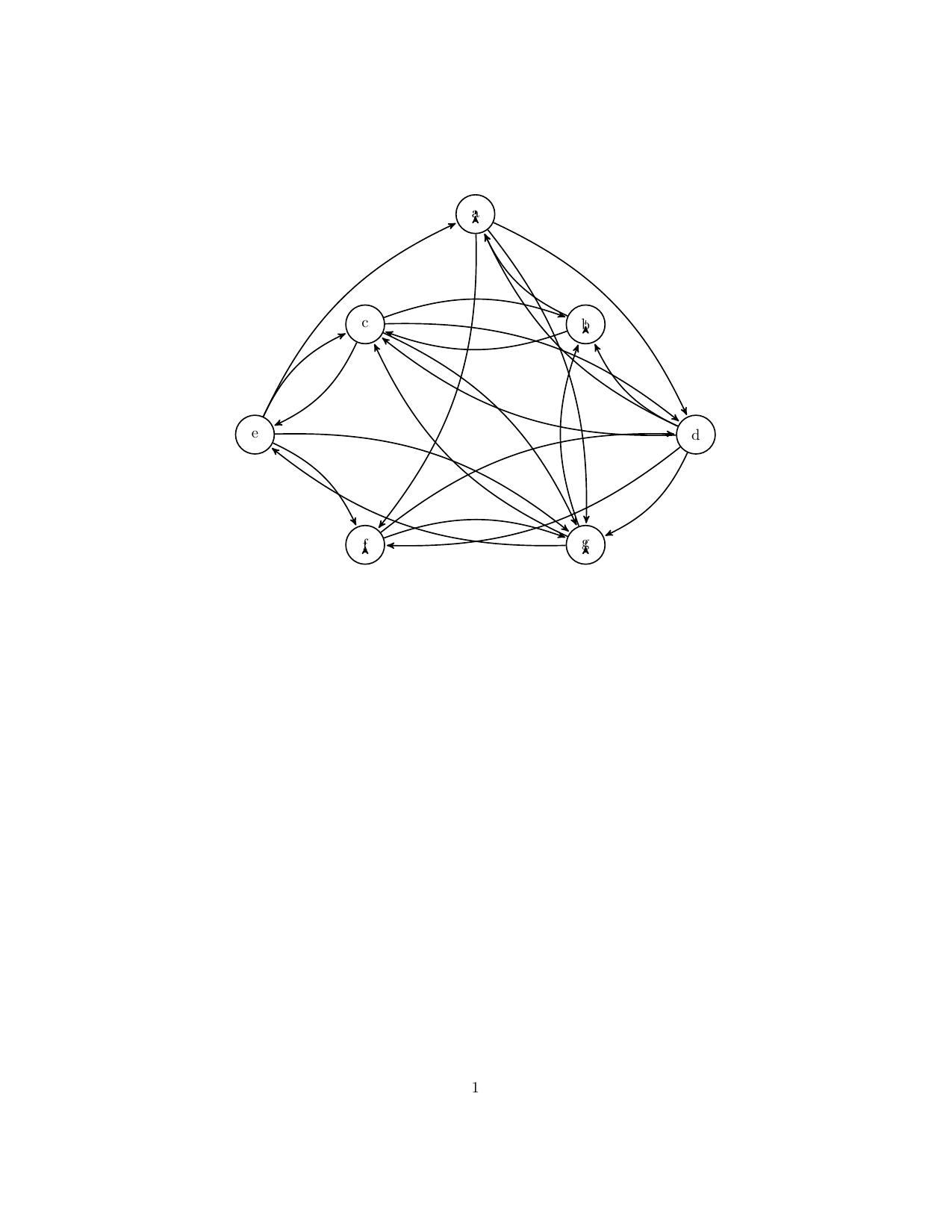
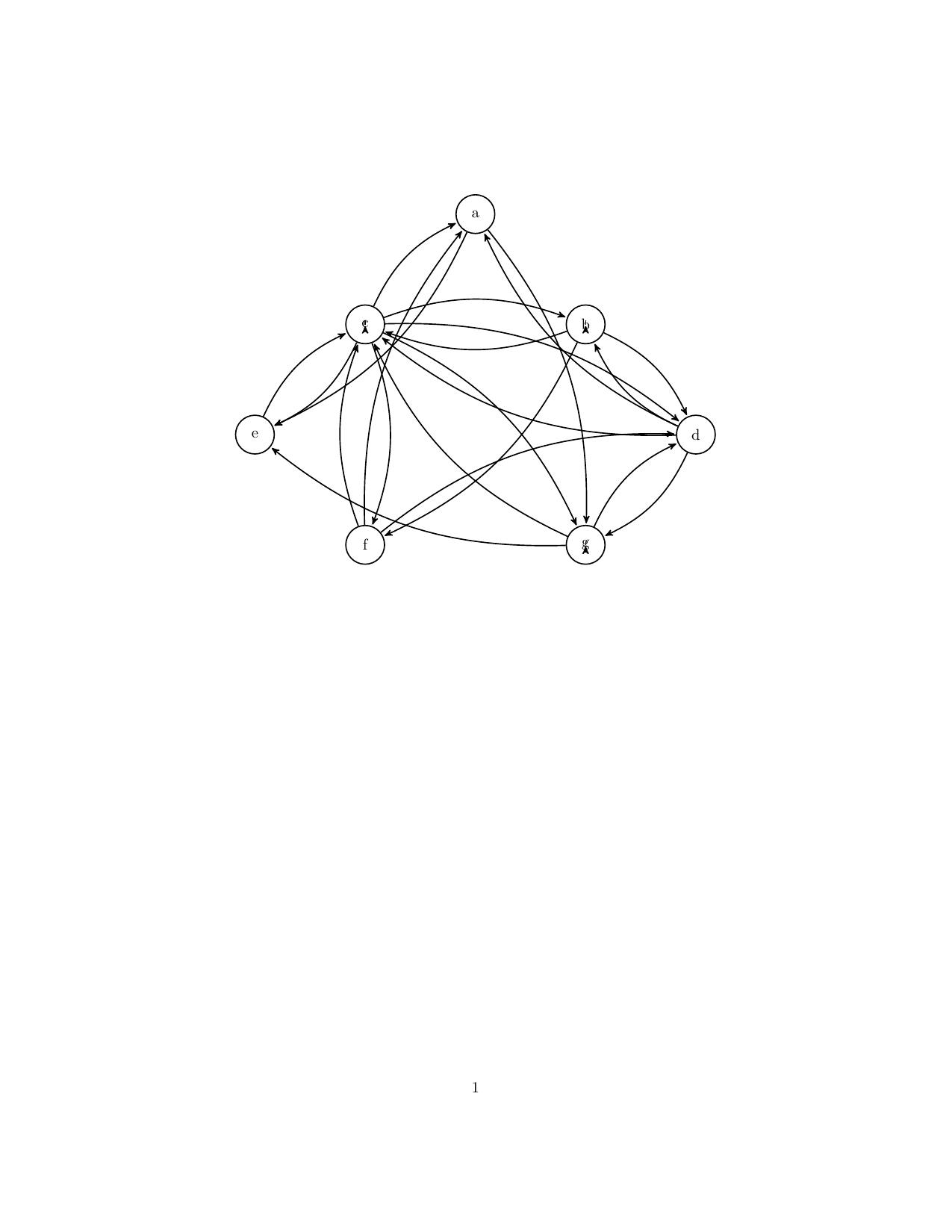
Code: Alles auswählen
#include <stdio.h>
#include <stdlib.h>
#include <time.h>
#include <string.h>
#define N 12
void generate_adjanzenzmatrix (int a [N][N], int n) {
int i, j;
for (i = 0; i < n; i++) {
for (j = 0; j < n; j++)
a [i][j] = rand () % 2;
}
}
void print_csv_adjanzenzmatrix (int a [N][N], int n) {
int i, j;
printf ("%c,", ' ');
for (i = 0; i < n-1; i++)
printf ("%c,", i + 'a');
printf ("%c\n", i+'a');
for (i = 0; i < n; i++) {
printf ("%c,", i+'a');
for (j = 0; j < n-1; j++) {
printf ("%c,", a [i][j] + '0');
}
printf ("%c\n", a [i][j] + '0');
}
}
void read_csv_adjanzenzmatrix (int a [N][N], int n) {
int i, j;
int ch;
scanf ("%c,", &ch);
for (i = 0; i < n-1; i++) {
scanf ("%c,", &ch);
}
scanf ("%c\n", &ch);
for (i = 0; i < n; i++) {
scanf ("%c,", &ch);
for (j = 0; j < n-1; j++) {
scanf ("%i,", &ch);
a [i] [j] = ch;
}
scanf ("%i\n", &ch);
a [i] [j] = ch;
}
}
void convert_csv_adjanzenzmatrix_to_adjazensliste (int a [N][N], int b [N][N], int n) {
int i, j, k;
for (i = 0; i < n; i++) {
for (j = 0, k = 0; j < n; j++) {
if (a [i][j] == 1) {
b [i][k] = j;
k++;
}
}
for (; k < n; k++)
b [i][k] = -1;
}
}
void convert_csv_adjanzenzliste_to_adjazensmatrix (int a [N][N], int b [N][N], int n) {
int i, j;
for (i = 0; i < n; i++) {
for (j = 0; j < n; j++)
a [i][j] = 0;
}
for (i = 0; i < n; i++) {
for (j = 0; j < n; j++)
if (b [i][j] != -1)
a [i][b[i][j]] = 1;
}
}
void read_csv_adjanzenzliste (int b [N][N], int n) {
int i, j, k;
int ch = 0;
scanf ("%c,", &ch);
for (i = 0; i < n-1; i++) {
scanf ("%c,", &ch);
}
scanf ("%c\n", &ch);
for (i = 0; i < n; i++) {
scanf ("%c,", &ch);
for (j = 0, k = 0; j < n-1; j++) {
scanf ("%c,", &ch);
if (ch == ' ')
b [i][j] = -1;
else
b [i][j] = ch - 'a';
}
scanf ("%c\n", &ch);
if (ch == ' ')
b [i][j] = -1;
else
b [i][j] = ch - 'a';
}
}
void print_csv_adjanzenzliste (int b [N][N], int n) {
int i, j;
printf ("%c,", ' ');
for (i = 0; i < n-1; i++)
printf ("%c,", i + 'a');
printf ("%c\n", i+'a');
for (i = 0; i < n; i++) {
printf ("%c,", i+'a');
for (j = 0; j < n-1; j++) {
if (b [i][j] != -1)
printf ("%c,", b [i][j] + 'a');
else
printf (" ,");
}
if (b [i][j] != -1)
printf ("%c\n", b [i][j] + 'a');
else
printf (" \n");
}
}
void print_tex (int a [N][N]) {
int i, j;
int codez = 8;
printf("\\documentclass{article}\n");
printf("\\usepackage[utf8]{inputenc}\n");
printf("\\usepackage{pgf, tikz}\n");
printf("\\usetikzlibrary{arrows , automata , positioning}\n");
printf("\\begin{document}\n\n");
printf("\\begin{center}\n");
printf("\\begin{tikzpicture}[>=stealth',shorten >=1pt,auto,node distance=2.5cm]\n");
printf("\%Knoten\n");
printf("\\node (a) [state, thick] {a};\n");
printf("\\node (b) [state, thick, right of= a, below of=a] {b};\n");
printf("\\node (c) [state, thick, left of= a, below of=a] {c};\n");
printf("\\node (d) [state, thick, right of= b, below of=b] {d};\n\n");
printf("\\node (e) [state, thick, left of= b, below of=b] {e};\n\n");
printf("\\node (g) [state, thick, left of=c, below of=c] {g};\n\n");
printf("\\node (f) [state, thick, below of=d] {f};\n\n");
printf("\\node (h) [state, thick, below of=e] {h};\n\n");
printf("\\node (i) [state, thick, below of=g] {i};\n\n");
printf("\\node (j) [state, thick, below of=f, left of=h] {f};\n\n");
printf("\\node (k) [state, thick, below of=h, right of=h] {h};\n\n");
printf("\\node (l) [state, thick, below of=h] {l};\n\n");
printf("\%Verbindungen\n");
printf("\\path[thick,->]\n");
char *leftright [] = {"left", "right"};
char *abovebelow [] = {"above", "below"};
for (i = 0; i < N; i++) {
for (j = 0; j < N; j++) {
if (a [i] [j] == 1)
printf ("(%c) edge [bend angle=20, bend left,below] (%c)\n", (char)('a' + i), (char)('a' + j));
}
}
printf(";\n");
printf("\\end{tikzpicture}\n");
printf("\\end{center}\n");
printf("\\end{document}\n");
}
int main (int argc, char *argv []) {
time_t t;
int n = N;
int a [N][N];
int b [N][N];
srand ((unsigned) time (&t));
if (argc != 3) {
printf ("Wrong Parameter!\ng: generete generate_adjanzenzmatrix\nr: read_csv_adjanzenzmatrix\ns: read_csv_adjanzenzliste\n\np: print_csv_adjanzenzmatrix\nq: print_csv_adjanzenzliste");
return 1;
}
if (strcmp(argv [1], "r") == 0) {
read_csv_adjanzenzmatrix (a, n);
convert_csv_adjanzenzmatrix_to_adjazensliste (a, b, n);
}
else if (strcmp (argv [1], "g") == 0) {
generate_adjanzenzmatrix (a, n);
convert_csv_adjanzenzmatrix_to_adjazensliste (a, b, n);
}
else if (strcmp (argv [1], "s") == 0) {
read_csv_adjanzenzliste (b, n);
convert_csv_adjanzenzliste_to_adjazensmatrix (a, b, n);
}
else
printf ("Wrong Parameter!\ng: generete generate_adjanzenzmatrix\nr: read_csv_adjanzenzmatrix\ns: read_csv_adjanzenzliste\n\np: print_csv_adjanzenzmatrix\nq: print_csv_adjanzenzliste");
if (strcmp (argv [2], "p") == 0)
print_csv_adjanzenzmatrix (a, n);
else if (strcmp (argv [2], "q") == 0)
print_csv_adjanzenzliste (b, n);
else if (strcmp (argv [2], "t") == 0)
print_tex (a);
return 0;
}
Code: Alles auswählen
#include <stdio.h>
#include <stdlib.h>
#include <time.h>
#include <string.h>
#define N 12
void generate_adjanzenzmatrix (int a [N][N], int n) {
int i, j;
for (i = 0; i < n; i++) {
for (j = 0; j < n; j++)
a [i][j] = rand () % 2;
}
}
void print_csv_adjanzenzmatrix (int a [N][N], int n) {
int i, j;
printf ("%c,", ' ');
for (i = 0; i < n-1; i++)
printf ("%c,", i + 'a');
printf ("%c\n", i+'a');
for (i = 0; i < n; i++) {
printf ("%c,", i+'a');
for (j = 0; j < n-1; j++) {
printf ("%c,", a [i][j] + '0');
}
printf ("%c\n", a [i][j] + '0');
}
}
void read_csv_adjanzenzmatrix (int a [N][N], int n) {
int i, j;
int ch;
scanf ("%c,", &ch);
for (i = 0; i < n-1; i++) {
scanf ("%c,", &ch);
}
scanf ("%c\n", &ch);
for (i = 0; i < n; i++) {
scanf ("%c,", &ch);
for (j = 0; j < n-1; j++) {
scanf ("%i,", &ch);
a [i] [j] = ch;
}
scanf ("%i\n", &ch);
a [i] [j] = ch;
}
}
void convert_csv_adjanzenzmatrix_to_adjazensliste (int a [N][N], int b [N][N], int n) {
int i, j, k;
for (i = 0; i < n; i++) {
for (j = 0, k = 0; j < n; j++) {
if (a [i][j] == 1) {
b [i][k] = j;
k++;
}
}
for (; k < n; k++)
b [i][k] = -1;
}
}
void convert_csv_adjanzenzliste_to_adjazensmatrix (int a [N][N], int b [N][N], int n) {
int i, j;
for (i = 0; i < n; i++) {
for (j = 0; j < n; j++)
a [i][j] = 0;
}
for (i = 0; i < n; i++) {
for (j = 0; j < n; j++)
if (b [i][j] != -1)
a [i][b[i][j]] = 1;
}
}
void read_csv_adjanzenzliste (int b [N][N], int n) {
int i, j, k;
int ch = 0;
scanf ("%c,", &ch);
for (i = 0; i < n-1; i++) {
scanf ("%c,", &ch);
}
scanf ("%c\n", &ch);
for (i = 0; i < n; i++) {
scanf ("%c,", &ch);
for (j = 0, k = 0; j < n-1; j++) {
scanf ("%c,", &ch);
if (ch == ' ')
b [i][j] = -1;
else
b [i][j] = ch - 'a';
}
scanf ("%c\n", &ch);
if (ch == ' ')
b [i][j] = -1;
else
b [i][j] = ch - 'a';
}
}
void print_csv_adjanzenzliste (int b [N][N], int n) {
int i, j;
printf ("%c,", ' ');
for (i = 0; i < n-1; i++)
printf ("%c,", i + 'a');
printf ("%c\n", i+'a');
for (i = 0; i < n; i++) {
printf ("%c,", i+'a');
for (j = 0; j < n-1; j++) {
if (b [i][j] != -1)
printf ("%c,", b [i][j] + 'a');
else
printf (" ,");
}
if (b [i][j] != -1)
printf ("%c\n", b [i][j] + 'a');
else
printf (" \n");
}
}
void print_tex (int a [N][N]) {
int i, j;
int codez = 8;
printf("\\documentclass{article}\n");
printf("\\usepackage[utf8]{inputenc}\n");
printf("\\usepackage{pgf, tikz}\n");
printf("\\usetikzlibrary{arrows , automata , positioning}\n");
printf("\\begin{document}\n\n");
printf("\\begin{center}\n");
printf("\\begin{tikzpicture}[>=stealth',shorten >=1pt,auto,node distance=2.5cm]\n");
printf("\%Knoten\n");
printf("\\node (a) [state, thick] {a};\n");
printf("\\node (b) [state, thick, right of= a, below of=a] {b};\n");
printf("\\node (c) [state, thick, left of= a, below of=a] {c};\n");
printf("\\node (d) [state, thick, below of=b, right of=b] {d};\n\n");
printf("\\node (e) [state, thick, below of=c, left of=c] {e};\n\n");
printf("\\node (g) [state, thick, below of=d] {g};\n\n");
printf("\\node (f) [state, thick, below of=e] {f};\n\n");
printf("\\node (h) [state, thick, below of=g] {h};\n\n");
printf("\\node (i) [state, thick, below of=f] {i};\n\n");
printf("\\node (j) [state, thick, below of=h, right of=i] {j};\n\n");
printf("\\node (k) [state, thick, right of=j] {k};\n\n");
printf("\\node (l) [state, thick, right of=k] {l};\n\n");
printf("\%Verbindungen\n");
printf("\\path[thick,->]\n");
char *leftright [] = {"left", "right"};
char *abovebelow [] = {"above", "below"};
for (i = 0; i < N; i++) {
for (j = 0; j < N; j++) {
if (a [i] [j] == 1)
printf ("(%c) edge [bend angle=20, bend left,below] (%c)\n", (char)('a' + i), (char)('a' + j));
}
}
printf(";\n");
printf("\\end{tikzpicture}\n");
printf("\\end{center}\n");
printf("\\end{document}\n");
}
int main (int argc, char *argv []) {
time_t t;
int n = N;
int a [N][N];
int b [N][N];
srand ((unsigned) time (&t));
if (argc != 3) {
printf ("Wrong Parameter!\ng: generete generate_adjanzenzmatrix\nr: read_csv_adjanzenzmatrix\ns: read_csv_adjanzenzliste\n\np: print_csv_adjanzenzmatrix\nq: print_csv_adjanzenzliste");
return 1;
}
if (strcmp(argv [1], "r") == 0) {
read_csv_adjanzenzmatrix (a, n);
convert_csv_adjanzenzmatrix_to_adjazensliste (a, b, n);
}
else if (strcmp (argv [1], "g") == 0) {
generate_adjanzenzmatrix (a, n);
convert_csv_adjanzenzmatrix_to_adjazensliste (a, b, n);
}
else if (strcmp (argv [1], "s") == 0) {
read_csv_adjanzenzliste (b, n);
convert_csv_adjanzenzliste_to_adjazensmatrix (a, b, n);
}
else
printf ("Wrong Parameter!\ng: generete generate_adjanzenzmatrix\nr: read_csv_adjanzenzmatrix\ns: read_csv_adjanzenzliste\n\np: print_csv_adjanzenzmatrix\nq: print_csv_adjanzenzliste");
if (strcmp (argv [2], "p") == 0)
print_csv_adjanzenzmatrix (a, n);
else if (strcmp (argv [2], "q") == 0)
print_csv_adjanzenzliste (b, n);
else if (strcmp (argv [2], "t") == 0)
print_tex (a);
return 0;
}
Code: Alles auswählen
#include <stdio.h>
#include <stdlib.h>
#include <time.h>
#include <string.h>
#define N 7
void generate_adjanzenzmatrix (int a [N][N], int n) {
int i, j;
for (i = 0; i < n; i++) {
for (j = 0; j < n; j++)
a [i][j] = rand () % 2;
}
}
void print_csv_adjanzenzmatrix (int a [N][N], int n) {
int i, j;
printf ("%c,", ' ');
for (i = 0; i < n-1; i++)
printf ("%c,", i + 'a');
printf ("%c\n", i+'a');
for (i = 0; i < n; i++) {
printf ("%c,", i+'a');
for (j = 0; j < n-1; j++) {
printf ("%c,", a [i][j] + '0');
}
printf ("%c\n", a [i][j] + '0');
}
}
void read_csv_adjanzenzmatrix (int a [N][N], int n) {
int i, j;
int ch;
scanf ("%c,", &ch);
for (i = 0; i < n-1; i++) {
scanf ("%c,", &ch);
}
scanf ("%c\n", &ch);
for (i = 0; i < n; i++) {
scanf ("%c,", &ch);
for (j = 0; j < n-1; j++) {
scanf ("%i,", &ch);
a [i] [j] = ch;
}
scanf ("%i\n", &ch);
a [i] [j] = ch;
}
}
void convert_csv_adjanzenzmatrix_to_adjazensliste (int a [N][N], int b [N][N], int n) {
int i, j, k;
for (i = 0; i < n; i++) {
for (j = 0, k = 0; j < n; j++) {
if (a [i][j] == 1) {
b [i][k] = j;
k++;
}
}
for (; k < n; k++)
b [i][k] = -1;
}
}
void convert_csv_adjanzenzliste_to_adjazensmatrix (int a [N][N], int b [N][N], int n) {
int i, j;
for (i = 0; i < n; i++) {
for (j = 0; j < n; j++)
a [i][j] = 0;
}
for (i = 0; i < n; i++) {
for (j = 0; j < n; j++)
if (b [i][j] != -1)
a [i][b[i][j]] = 1;
}
}
void read_csv_adjanzenzliste (int b [N][N], int n) {
int i, j, k;
int ch = 0;
scanf ("%c,", &ch);
for (i = 0; i < n-1; i++) {
scanf ("%c,", &ch);
}
scanf ("%c\n", &ch);
for (i = 0; i < n; i++) {
scanf ("%c,", &ch);
for (j = 0, k = 0; j < n-1; j++) {
scanf ("%c,", &ch);
if (ch == ' ')
b [i][j] = -1;
else
b [i][j] = ch - 'a';
}
scanf ("%c\n", &ch);
if (ch == ' ')
b [i][j] = -1;
else
b [i][j] = ch - 'a';
}
}
void print_csv_adjanzenzliste (int b [N][N], int n) {
int i, j;
printf ("%c,", ' ');
for (i = 0; i < n-1; i++)
printf ("%c,", i + 'a');
printf ("%c\n", i+'a');
for (i = 0; i < n; i++) {
printf ("%c,", i+'a');
for (j = 0; j < n-1; j++) {
if (b [i][j] != -1)
printf ("%c,", b [i][j] + 'a');
else
printf (" ,");
}
if (b [i][j] != -1)
printf ("%c\n", b [i][j] + 'a');
else
printf (" \n");
}
}
void print_tex (int a [N][N]) {
int i, j;
int codez = 8;
printf("\\documentclass{article}\n");
printf("\\usepackage[utf8]{inputenc}\n");
printf("\\usepackage{pgf, tikz}\n");
printf("\\usetikzlibrary{arrows , automata , positioning}\n");
printf("\\begin{document}\n\n");
printf("\\begin{center}\n");
printf("\\begin{tikzpicture}[>=stealth',shorten >=1pt,auto,node distance=2.5cm]\n");
printf("\%Knoten\n");
printf("\\node (a) [state, thick] {a};\n");
printf("\\node (b) [state, thick, right of= a, below of=a] {b};\n");
printf("\\node (c) [state, thick, left of= a, below of=a] {c};\n");
printf("\\node (d) [state, thick, below of=b, right of=b] {d};\n\n");
printf("\\node (e) [state, thick, below of=c, left of=c] {e};\n\n");
printf("\\node (g) [state, thick, below of=d, left of=d] {g};\n\n");
printf("\\node (f) [state, thick, below of=e, right of=e] {f};\n\n");
printf("\%Verbindungen\n");
printf("\\path[thick,->]\n");
char *leftright [] = {"left", "right"};
char *abovebelow [] = {"above", "below"};
for (i = 0; i < N; i++) {
for (j = 0; j < N; j++) {
if (a [i] [j] == 1)
printf ("(%c) edge [bend angle=20, bend left,below] (%c)\n", (char)('a' + i), (char)('a' + j));
}
}
printf(";\n");
printf("\\end{tikzpicture}\n");
printf("\\end{center}\n");
printf("\\end{document}\n");
}
int main (int argc, char *argv []) {
time_t t;
int n = N;
int a [N][N];
int b [N][N];
srand ((unsigned) time (&t));
if (argc != 3) {
printf ("Wrong Parameter!\ng: generete generate_adjanzenzmatrix\nr: read_csv_adjanzenzmatrix\ns: read_csv_adjanzenzliste\n\np: print_csv_adjanzenzmatrix\nq: print_csv_adjanzenzliste");
return 1;
}
if (strcmp(argv [1], "r") == 0) {
read_csv_adjanzenzmatrix (a, n);
convert_csv_adjanzenzmatrix_to_adjazensliste (a, b, n);
}
else if (strcmp (argv [1], "g") == 0) {
generate_adjanzenzmatrix (a, n);
convert_csv_adjanzenzmatrix_to_adjazensliste (a, b, n);
}
else if (strcmp (argv [1], "s") == 0) {
read_csv_adjanzenzliste (b, n);
convert_csv_adjanzenzliste_to_adjazensmatrix (a, b, n);
}
else
printf ("Wrong Parameter!\ng: generete generate_adjanzenzmatrix\nr: read_csv_adjanzenzmatrix\ns: read_csv_adjanzenzliste\n\np: print_csv_adjanzenzmatrix\nq: print_csv_adjanzenzliste");
if (strcmp (argv [2], "p") == 0)
print_csv_adjanzenzmatrix (a, n);
else if (strcmp (argv [2], "q") == 0)
print_csv_adjanzenzliste (b, n);
else if (strcmp (argv [2], "t") == 0)
print_tex (a);
return 0;
}
Code: Alles auswählen
#include <stdio.h>
#include <stdlib.h>
#include <time.h>
#include <string.h>
#define N 7
int Q [1024];
int Qtop = 0;
int Qbottom = 0;
void Qput (int v) {
Q [Qtop] = v;
Qtop ++;
}
int Qget (void) {
int v = Q [Qbottom];
Qbottom++;
return v;
}
int QIsNotEmpty () {
return (Qbottom < Qtop);
}
void breadth_first_search (int a [N][N], int *component, int r) {
int pred [1024];
int w, v;
int i = 0;
for (i = 0; i < 1024; i++) {
pred [i] = -1;
component [i] = -1;
}
pred [r] = r;
component [r] = r;
Qput (r);
while (QIsNotEmpty ()) {
v = Qget ();
printf ("%c\n", v + 'a');
for (w = 0; w < N; w++) {
if (a [v][w]) {
if (pred [w] == -1) {
pred [w] = v;
component [w] = component [v];
Qput (w);
}
}
}
}
}
int _bfs (int a [N][N], int marked [N], int r) {
int i;
int retv;
if (marked [r] == -1) {
marked [r] = 1;
for (i = 0; i < N; i++)
if(retv = _bfs (a, marked, a [r][i]))
printf ("%c", r+'a');
return retv;
}
return 0;
}
void bfs (int a [N][N], int r) {
int i;
int marked [N];
for (i = 0; i < N; i++)
marked [i] = -1;
_bfs (a, marked, r);
}
void generate_adjanzenzmatrix (int a [N][N], int n) {
int i, j;
for (i = 0; i < n; i++) {
for (j = 0; j < n; j++) {
a [i][j] = -1;
}
}
for (i = 0; i < n; i++) {
for (j = 0; j < n; j++) {
if ((a [i][j] == -1) && (a [j][i] == -1)) {
a[j][i] = a [i][j] = rand () % 2;
}
}
}
}
void print_csv_adjanzenzmatrix (int a [N][N], int n) {
int i, j;
printf ("%c,", ' ');
for (i = 0; i < n-1; i++)
printf ("%c,", i + 'a');
printf ("%c\n", i+'a');
for (i = 0; i < n; i++) {
printf ("%c,", i+'a');
for (j = 0; j < n-1; j++) {
printf ("%c,", a [i][j] + '0');
}
printf ("%c\n", a [i][j] + '0');
}
}
void read_csv_adjanzenzmatrix (int a [N][N], int n) {
int i, j;
int ch;
scanf ("%c,", &ch);
for (i = 0; i < n-1; i++) {
scanf ("%c,", &ch);
}
scanf ("%c\n", &ch);
for (i = 0; i < n; i++) {
scanf ("%c,", &ch);
for (j = 0; j < n-1; j++) {
scanf ("%i,", &ch);
a [i] [j] = ch;
}
scanf ("%i\n", &ch);
a [i] [j] = ch;
}
}
void convert_csv_adjanzenzmatrix_to_adjazensliste (int a [N][N], int b [N][N], int n) {
int i, j, k;
for (i = 0; i < n; i++) {
for (j = 0, k = 0; j < n; j++) {
if (a [i][j] == 1) {
b [i][k] = j;
k++;
}
}
for (; k < n; k++)
b [i][k] = -1;
}
}
void convert_csv_adjanzenzliste_to_adjazensmatrix (int a [N][N], int b [N][N], int n) {
int i, j;
for (i = 0; i < n; i++) {
for (j = 0; j < n; j++)
a [i][j] = 0;
}
for (i = 0; i < n; i++) {
for (j = 0; j < n; j++)
if (b [i][j] != -1)
a [i][b[i][j]] = 1;
}
}
void read_csv_adjanzenzliste (int b [N][N], int n) {
int i, j, k;
int ch = 0;
scanf ("%c,", &ch);
for (i = 0; i < n-1; i++) {
scanf ("%c,", &ch);
}
scanf ("%c\n", &ch);
for (i = 0; i < n; i++) {
scanf ("%c,", &ch);
for (j = 0, k = 0; j < n-1; j++) {
scanf ("%c,", &ch);
if (ch == ' ')
b [i][j] = -1;
else
b [i][j] = ch - 'a';
}
scanf ("%c\n", &ch);
if (ch == ' ')
b [i][j] = -1;
else
b [i][j] = ch - 'a';
}
}
void print_csv_adjanzenzliste (int b [N][N], int n) {
int i, j;
printf ("%c,", ' ');
for (i = 0; i < n-1; i++)
printf ("%c,", i + 'a');
printf ("%c\n", i+'a');
for (i = 0; i < n; i++) {
printf ("%c,", i+'a');
for (j = 0; j < n-1; j++) {
if (b [i][j] != -1)
printf ("%c,", b [i][j] + 'a');
else
printf (" ,");
}
if (b [i][j] != -1)
printf ("%c\n", b [i][j] + 'a');
else
printf (" \n");
}
}
void print_tex (int a [N][N], int component [1024]) {
int i, j;
int codez = 8;
printf("\\documentclass{article}\n");
printf("\\usepackage[utf8]{inputenc}\n");
printf("\\usepackage{pgf, tikz}\n");
printf("\\usetikzlibrary{arrows , automata , positioning}\n");
printf("\\begin{document}\n\n");
printf("\\begin{center}\n");
printf("\\begin{tikzpicture}[>=stealth',shorten >=1pt,auto,node distance=2.5cm]\n");
printf("\%Knoten\n");
printf("\\node (a) [state, thick] {a};\n");
printf("\\node (b) [state, thick, right of= a, below of=a] {b};\n");
printf("\\node (c) [state, thick, left of= a, below of=a] {c};\n");
printf("\\node (d) [state, thick, below of=b, right of=b] {d};\n\n");
printf("\\node (e) [state, thick, below of=c, left of=c] {e};\n\n");
printf("\\node (g) [state, thick, below of=d, left of=d] {g};\n\n");
printf("\\node (f) [state, thick, below of=e, right of=e] {f};\n\n");
printf("\%Verbindungen\n");
printf("\\path[thick,->]\n");
char *leftright [] = {"left", "right"};
char *abovebelow [] = {"above", "below"};
for (i = 0; i < N; i++) {
for (j = 0; j < N; j++) {
if (a [i] [j] == 1)
printf ("(%c) edge [bend angle=20, bend left,below] (%c)\n", (char)('a' + i), (char)('a' + j));
}
}
for (i = 0; i < N-1; i++)
printf ("(%c) edge [bend angle=20, bend left,below, style thick] (%c)\n", component [i] + 'a', component [i+1] + 'a');
printf(";\n");
printf("\\end{tikzpicture}\n");
printf("\\end{center}\n");
printf("\\end{document}\n");
}
int main (int argc, char *argv []) {
time_t t;
int n = N;
int a [N][N];
int b [N][N];
int component [1024];
srand ((unsigned) time (&t));
if (argc != 3) {
printf ("Wrong Parameter!\ng: generete generate_adjanzenzmatrix\nr: read_csv_adjanzenzmatrix\ns: read_csv_adjanzenzliste\n\np: print_csv_adjanzenzmatrix\nq: print_csv_adjanzenzliste");
return 1;
}
if (strcmp(argv [1], "r") == 0) {
read_csv_adjanzenzmatrix (a, n);
convert_csv_adjanzenzmatrix_to_adjazensliste (a, b, n);
}
else if (strcmp (argv [1], "g") == 0) {
generate_adjanzenzmatrix (a, n);
convert_csv_adjanzenzmatrix_to_adjazensliste (a, b, n);
}
else if (strcmp (argv [1], "s") == 0) {
read_csv_adjanzenzliste (b, n);
convert_csv_adjanzenzliste_to_adjazensmatrix (a, b, n);
}
else
printf ("Wrong Parameter!\ng: generete generate_adjanzenzmatrix\nr: read_csv_adjanzenzmatrix\ns: read_csv_adjanzenzliste\n\np: print_csv_adjanzenzmatrix\nq: print_csv_adjanzenzliste");
bfs (a, 0);
if (strcmp (argv [2], "p") == 0)
print_csv_adjanzenzmatrix (a, n);
else if (strcmp (argv [2], "q") == 0)
print_csv_adjanzenzliste (b, n);
else if (strcmp (argv [2], "t") == 0)
print_tex (a, component);
return 0;
}
Code: Alles auswählen
\documentclass{article}
\usepackage[utf8]{inputenc}
\usepackage{pgf, tikz}
\usetikzlibrary{arrows , automata , positioning}
\begin{document}
\begin{center}
\begin{tikzpicture}[>=stealth',shorten >=1pt,auto,node distance=2.5cm]
%Knoten
\node (a) [state, thick] {a};
\node (b) [state, thick, right of= a, below of=a] {b};
\node (c) [state, thick, left of= a, below of=a] {c};
\node (d) [state, thick, below of=b, right of=b] {d};
\node (e) [state, thick, below of=c, left of=c] {e};
\node (g) [state, thick, below of=d, left of=d] {g};
\node (f) [state, thick, below of=e, right of=e] {f};
%Verbindungen
\path[thick,->]
(a) edge [bend angle=20, bend left,below] (c)
(a) edge [bend angle=20, bend left,below] (d)
(a) edge [bend angle=20, bend left,below] (e)
(a) edge [bend angle=20, bend left,below] (g)
(b) edge [bend angle=20, bend left,below] (c)
(b) edge [bend angle=20, bend left,below] (d)
(b) edge [bend angle=20, bend left,below] (g)
(c) edge [bend angle=20, bend left,below] (a)
(c) edge [bend angle=20, bend left,below] (b)
(c) edge [bend angle=20, bend left,below] (e)
(c) edge [bend angle=20, bend left,below] (f)
(d) edge [bend angle=20, bend left,below] (a)
(d) edge [bend angle=20, bend left,below] (d)
(d) edge [bend angle=20, bend left,below] (f)
(e) edge [bend angle=20, bend left,below] (a)
(e) edge [bend angle=20, bend left,below] (d)
(e) edge [bend angle=20, bend left,below] (f)
(f) edge [bend angle=20, bend left,below] (a)
(f) edge [bend angle=20, bend left,below] (b)
(f) edge [bend angle=20, bend left,below] (c)
(f) edge [bend angle=20, bend left,below] (e)
(f) edge [bend angle=20, bend left,below] (g)
(g) edge [bend angle=20, bend left,below] (a)
(g) edge [bend angle=20, bend left,below] (b)
(g) edge [bend angle=20, bend left,below] (d)
(g) edge [bend angle=20, bend left,below] (f)
(g) edge [bend angle=20, bend left,below] (g)
;
\end{tikzpicture}
\end{center}
\end{document}
Code: Alles auswählen
\documentclass{article}
\usepackage[utf8]{inputenc}
\usepackage{pgf, tikz}
\usetikzlibrary{arrows , automata , positioning}
\begin{document}
\begin{center}
\begin{tikzpicture}[>=stealth',shorten >=1pt,auto,node distance=2.5cm]
%Knoten
\node (a) [state, thick] {a};
\node (b) [state, thick, right of= a, below of=a] {b};
\node (c) [state, thick, left of= a, below of=a] {c};
\node (d) [state, thick, right of= b, below of=b] {d};
\node (e) [state, thick, left of= b, below of=b] {e};
\node (g) [state, thick, left of=c, below of=c] {g};
\node (f) [state, thick, below of=d] {f};
\node (h) [state, thick, below of=e] {h};
\node (i) [state, thick, below of=g] {i};
\node (j) [state, thick, below of=f, left of=h] {f};
\node (k) [state, thick, below of=h, right of=h] {h};
\node (l) [state, thick, below of=h] {l};
%Verbindungen
\path[thick,->]
(a) edge [bend angle=20] (f)
(a) edge [bend angle=20] (g)
(a) edge [bend angle=20] (i)
(a) edge [bend angle=20] (k)
(a) edge [bend angle=20] (l)
(b) edge [bend angle=20] (c)
(b) edge [bend angle=20] (d)
(b) edge [bend angle=20] (e)
(b) edge [bend angle=20] (f)
(b) edge [bend angle=20] (h)
(b) edge [bend angle=20] (k)
(b) edge [bend angle=20] (l)
(c) edge [bend angle=20] (a)
(c) edge [bend angle=20] (b)
(c) edge [bend angle=20] (c)
(c) edge [bend angle=20] (h)
(c) edge [bend angle=20] (k)
(c) edge [bend angle=20] (l)
(d) edge [bend angle=20] (b)
(d) edge [bend angle=20] (c)
(d) edge [bend angle=20] (d)
(d) edge [bend angle=20] (e)
(d) edge [bend angle=20] (h)
(d) edge [bend angle=20] (l)
(e) edge [bend angle=20] (a)
(e) edge [bend angle=20] (b)
(e) edge [bend angle=20] (d)
(e) edge [bend angle=20] (e)
(e) edge [bend angle=20] (f)
(e) edge [bend angle=20] (g)
(e) edge [bend angle=20] (h)
(e) edge [bend angle=20] (k)
(e) edge [bend angle=20] (l)
(f) edge [bend angle=20] (b)
(f) edge [bend angle=20] (e)
(f) edge [bend angle=20] (f)
(f) edge [bend angle=20] (g)
(f) edge [bend angle=20] (h)
(f) edge [bend angle=20] (l)
(g) edge [bend angle=20] (b)
(g) edge [bend angle=20] (e)
(g) edge [bend angle=20] (f)
(g) edge [bend angle=20] (k)
(g) edge [bend angle=20] (l)
(h) edge [bend angle=20] (f)
(h) edge [bend angle=20] (g)
(h) edge [bend angle=20] (j)
(h) edge [bend angle=20] (k)
(i) edge [bend angle=20] (a)
(i) edge [bend angle=20] (c)
(i) edge [bend angle=20] (d)
(i) edge [bend angle=20] (e)
(i) edge [bend angle=20] (g)
(i) edge [bend angle=20] (h)
(i) edge [bend angle=20] (i)
(i) edge [bend angle=20] (j)
(j) edge [bend angle=20] (f)
(j) edge [bend angle=20] (k)
(k) edge [bend angle=20] (c)
(k) edge [bend angle=20] (h)
(k) edge [bend angle=20] (l)
(l) edge [bend angle=20] (b)
(l) edge [bend angle=20] (c)
(l) edge [bend angle=20] (d)
(l) edge [bend angle=20] (e)
(l) edge [bend angle=20] (f)
(l) edge [bend angle=20] (g)
(l) edge [bend angle=20] (h)
(l) edge [bend angle=20] (i)
(l) edge [bend angle=20] (j)
(l) edge [bend angle=20] (l)
;
\end{tikzpicture}
\end{center}
\end{document}
Code: Alles auswählen
\documentclass{article}
\usepackage[utf8]{inputenc}
\usepackage{pgf, tikz}
\usetikzlibrary{arrows , automata , positioning}
\begin{document}
\begin{center}
\begin{tikzpicture}[>=stealth',shorten >=1pt,auto,node distance=2.5cm]
%Knoten
\node (a) [state, thick] {a};
\node (b) [state, thick, right of= a, below of=a] {b};
\node (c) [state, thick, left of= a, below of=a] {c};
\node (d) [state, thick, below of=b, right of=b] {d};
\node (e) [state, thick, below of=c, left of=c] {e};
\node (g) [state, thick, below of=d, left of=d] {g};
\node (f) [state, thick, below of=e, right of=e] {f};
%Verbindungen
\path[thick,->]
(a) edge [bend angle=20, bend left,below] (a)
(a) edge [bend angle=20, bend left,below] (d)
(a) edge [bend angle=20, bend left,below] (f)
(a) edge [bend angle=20, bend left,below] (g)
(b) edge [bend angle=20, bend left,below] (a)
(b) edge [bend angle=20, bend left,below] (b)
(b) edge [bend angle=20, bend left,below] (c)
(c) edge [bend angle=20, bend left,below] (b)
(c) edge [bend angle=20, bend left,below] (d)
(c) edge [bend angle=20, bend left,below] (e)
(c) edge [bend angle=20, bend left,below] (g)
(d) edge [bend angle=20, bend left,below] (a)
(d) edge [bend angle=20, bend left,below] (b)
(d) edge [bend angle=20, bend left,below] (c)
(d) edge [bend angle=20, bend left,below] (f)
(d) edge [bend angle=20, bend left,below] (g)
(e) edge [bend angle=20, bend left,below] (a)
(e) edge [bend angle=20, bend left,below] (c)
(e) edge [bend angle=20, bend left,below] (f)
(e) edge [bend angle=20, bend left,below] (g)
(f) edge [bend angle=20, bend left,below] (d)
(f) edge [bend angle=20, bend left,below] (f)
(f) edge [bend angle=20, bend left,below] (g)
(g) edge [bend angle=20, bend left,below] (b)
(g) edge [bend angle=20, bend left,below] (c)
(g) edge [bend angle=20, bend left,below] (e)
(g) edge [bend angle=20, bend left,below] (g)
;
\end{tikzpicture}
\end{center}
\end{document}
3
5
6
1
2
4
a, `, `, `, `, `, `,
Code: Alles auswählen
\documentclass{article}
\usepackage[utf8]{inputenc}
\usepackage{pgf, tikz}
\usetikzlibrary{arrows , automata , positioning}
\begin{document}
\begin{center}
\begin{tikzpicture}[>=stealth',shorten >=1pt,auto,node distance=2.5cm]
%Knoten
\node (a) [state, thick] {a};
\node (b) [state, thick, right of= a, below of=a] {b};
\node (c) [state, thick, left of= a, below of=a] {c};
\node (d) [state, thick, below of=b, right of=b] {d};
\node (e) [state, thick, below of=c, left of=c] {e};
\node (g) [state, thick, below of=d, left of=d] {g};
\node (f) [state, thick, below of=e, right of=e] {f};
%Verbindungen
\path[thick,->]
(a) edge [bend angle=20, bend left,below] (b)
(a) edge [bend angle=20, bend left,below] (c)
(a) edge [bend angle=20, bend left,below] (d)
(b) edge [bend angle=20, bend left,below] (b)
(b) edge [bend angle=20, bend left,below] (d)
(b) edge [bend angle=20, bend left,below] (e)
(b) edge [bend angle=20, bend left,below] (f)
(c) edge [bend angle=20, bend left,below] (a)
(c) edge [bend angle=20, bend left,below] (d)
(c) edge [bend angle=20, bend left,below] (e)
(c) edge [bend angle=20, bend left,below] (f)
(d) edge [bend angle=20, bend left,below] (a)
(d) edge [bend angle=20, bend left,below] (b)
(d) edge [bend angle=20, bend left,below] (f)
(d) edge [bend angle=20, bend left,below] (g)
(e) edge [bend angle=20, bend left,below] (a)
(e) edge [bend angle=20, bend left,below] (b)
(e) edge [bend angle=20, bend left,below] (c)
(e) edge [bend angle=20, bend left,below] (d)
(e) edge [bend angle=20, bend left,below] (e)
(e) edge [bend angle=20, bend left,below] (f)
(f) edge [bend angle=20, bend left,below] (a)
(f) edge [bend angle=20, bend left,below] (b)
(f) edge [bend angle=20, bend left,below] (d)
(f) edge [bend angle=20, bend left,below] (f)
(g) edge [bend angle=20, bend left,below] (a)
(g) edge [bend angle=20, bend left,below] (c)
(g) edge [bend angle=20, bend left,below] (d)
(g) edge [bend angle=20, bend left,below] (e)
(g) edge [bend angle=20, bend left,below] (f)
;
\end{tikzpicture}
\end{center}
\end{document}
1
2
3
4
5
6
a, `, `, `, `, `, `,
Code: Alles auswählen
2
4
5
6
3
\documentclass{article}
\usepackage[utf8]{inputenc}
\usepackage{pgf, tikz}
\usetikzlibrary{arrows , automata , positioning}
\begin{document}
\begin{center}
\begin{tikzpicture}[>=stealth',shorten >=1pt,auto,node distance=2.5cm]
%Knoten
\node (a) [state, thick] {a};
\node (b) [state, thick, right of= a, below of=a] {b};
\node (c) [state, thick, left of= a, below of=a] {c};
\node (d) [state, thick, below of=b, right of=b] {d};
\node (e) [state, thick, below of=c, left of=c] {e};
\node (g) [state, thick, below of=d, left of=d] {g};
\node (f) [state, thick, below of=e, right of=e] {f};
%Verbindungen
\path[thick,->]
(a) edge [bend angle=20, bend left,below] (c)
(a) edge [bend angle=20, bend left,below] (e)
(a) edge [bend angle=20, bend left,below] (f)
(a) edge [bend angle=20, bend left,below] (g)
(b) edge [bend angle=20, bend left,below] (a)
(b) edge [bend angle=20, bend left,below] (d)
(b) edge [bend angle=20, bend left,below] (f)
(b) edge [bend angle=20, bend left,below] (g)
(c) edge [bend angle=20, bend left,below] (c)
(c) edge [bend angle=20, bend left,below] (g)
(d) edge [bend angle=20, bend left,below] (d)
(d) edge [bend angle=20, bend left,below] (e)
(d) edge [bend angle=20, bend left,below] (f)
(e) edge [bend angle=20, bend left,below] (a)
(e) edge [bend angle=20, bend left,below] (c)
(e) edge [bend angle=20, bend left,below] (d)
(e) edge [bend angle=20, bend left,below] (e)
(e) edge [bend angle=20, bend left,below] (f)
(e) edge [bend angle=20, bend left,below] (g)
(f) edge [bend angle=20, bend left,below] (c)
(f) edge [bend angle=20, bend left,below] (d)
(f) edge [bend angle=20, bend left,below] (e)
(f) edge [bend angle=20, bend left,below] (f)
(g) edge [bend angle=20, bend left,below] (a)
(g) edge [bend angle=20, bend left,below] (c)
(g) edge [bend angle=20, bend left,below] (d)
(g) edge [bend angle=20, bend left,below] (e)
(g) edge [bend angle=20, bend left,below] (f)
(g) edge [bend angle=20, bend left,below] (g)
(a) edge [bend angle=20, bend left,below, style thick] (`)
(`) edge [bend angle=20, bend left,below, style thick] (`)
(`) edge [bend angle=20, bend left,below, style thick] (`)
(`) edge [bend angle=20, bend left,below, style thick] (`)
(`) edge [bend angle=20, bend left,below, style thick] (`)
(`) edge [bend angle=20, bend left,below, style thick] (`)
;
\end{tikzpicture}
\end{center}
\end{document}
https://www.ituenix.de/nextcloud/data/d ... est2-1.jpg
https://www.ituenix.de/nextcloud/data/d ... /test2.aux
https://www.ituenix.de/nextcloud/data/d ... /test2.log
https://www.ituenix.de/nextcloud/data/d ... /test2.pdf
https://www.ituenix.de/nextcloud/data/d ... /test2.tex
https://www.ituenix.de/nextcloud/data/d ... est3-1.jpg
https://www.ituenix.de/nextcloud/data/d ... /test3.aux
https://www.ituenix.de/nextcloud/data/d ... /test3.log
https://www.ituenix.de/nextcloud/data/d ... /test3.pdf
https://www.ituenix.de/nextcloud/data/d ... /test3.tex
https://www.ituenix.de/nextcloud/data/d ... est4-1.jpg
https://www.ituenix.de/nextcloud/data/d ... /test4.aux
https://www.ituenix.de/nextcloud/data/d ... /test4.log
https://www.ituenix.de/nextcloud/data/d ... /test4.pdf
https://www.ituenix.de/nextcloud/data/d ... /test4.tex
https://www.ituenix.de/nextcloud/data/d ... /test5.aux
https://www.ituenix.de/nextcloud/data/d ... /test5.log
https://www.ituenix.de/nextcloud/data/d ... /test5.pdf
https://www.ituenix.de/nextcloud/data/d ... /test5.tex
https://www.ituenix.de/nextcloud/data/d ... s/test.aux
https://www.ituenix.de/nextcloud/data/d ... s/test.log
https://www.ituenix.de/nextcloud/data/d ... s/test.pdf
https://www.ituenix.de/nextcloud/data/d ... st.png.vcd
https://www.ituenix.de/nextcloud/data/d ... s/test.tex
https://www.ituenix.de/nextcloud/data/d ... egraphs2.c
https://www.ituenix.de/nextcloud/data/d ... egraphs3.c
https://www.ituenix.de/nextcloud/data/d ... egraphs4.c
https://www.ituenix.de/nextcloud/data/d ... tegraphs.c